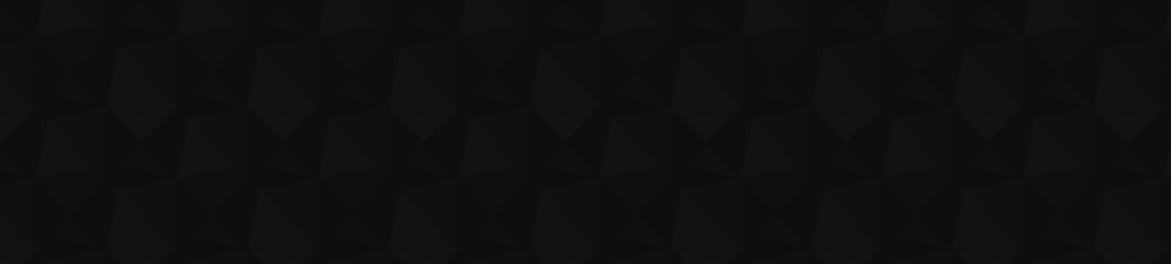
- 310
- 1 960 353
Ryan Noonan
United States
เข้าร่วมเมื่อ 5 ธ.ค. 2014
Programming Languages, Spreadsheet Tutorials, Data Analysis Tutorials, Data Visualization, Software Tips & Tricks, Tech Tutorials, Education
Python Puzzlers Series - Create Combination and Permutation Functions
Welcome back everybody.
Today, our python puzzler challenge is to create functions that can answer the following two questions:
1. Jerry needs to choose 3 friends from a group of 9 friends to be on his bobsled team. How many ways can the selection be made? Hint: Order is not important.
2. Seven cars enter a race. How many possibilities are there for the first through third place finishers? Hint: Order is important.
This puzzler is just to get the brain going and thinking about how to solve problems relating to combinations and permutations and the algorithms. There are probably more complicated but better ways to implement this in python.
If you are not concerned about the algorithm - for large numbers/calculations - I would suggest using a package such as math, scipy, and/or gmpy2 for combinations and permutations and NOT using the python formula implementation.
Examples:
import itertools
import math
import gmpy2
from scipy.special import comb
from scipy.special import perm
print(len(list(itertools.combinations(range(1,10),3))))
print(math.comb(9,3))
print(comb(9,3, exact=True))
print(gmpy2.comb(9,3))
print(len(list(itertools.permutations(range(1,8),3))))
print(math.perm(7,3))
print(perm(7,3, exact=True))
itertools.permutations with big numbers might not run.
Only the standard formulas are covered here. Does not include with replacement/with repetition, all possible, etc. Also, this function does not account for corner cases (error checking) like if you enter a larger choose number (r) than the total number (n), etc.
math.comb and math.perm are new in version 3.8.
Combinations are the number of different ways in which objects can be arranged without regard to order.
Permutations are the number of different ways in which objects can be arranged in order.
This puzzler level is easy.
In this series we will include: data structures, algorithms, brain teasers, puzzles, and coding challenges.
The purpose of these tutorials will be to:
* Help you improve your coding skills or knock off the rust.
* Help to improve your problem solving skills.
* Help you answer questions on sites like LeetCode, Codewars, HackerRank, etc.
* Help you prepare for interviews - if needed.
* And most importantly - have fun coding by solving brain teasers and puzzles.
Today, our python puzzler challenge is to create functions that can answer the following two questions:
1. Jerry needs to choose 3 friends from a group of 9 friends to be on his bobsled team. How many ways can the selection be made? Hint: Order is not important.
2. Seven cars enter a race. How many possibilities are there for the first through third place finishers? Hint: Order is important.
This puzzler is just to get the brain going and thinking about how to solve problems relating to combinations and permutations and the algorithms. There are probably more complicated but better ways to implement this in python.
If you are not concerned about the algorithm - for large numbers/calculations - I would suggest using a package such as math, scipy, and/or gmpy2 for combinations and permutations and NOT using the python formula implementation.
Examples:
import itertools
import math
import gmpy2
from scipy.special import comb
from scipy.special import perm
print(len(list(itertools.combinations(range(1,10),3))))
print(math.comb(9,3))
print(comb(9,3, exact=True))
print(gmpy2.comb(9,3))
print(len(list(itertools.permutations(range(1,8),3))))
print(math.perm(7,3))
print(perm(7,3, exact=True))
itertools.permutations with big numbers might not run.
Only the standard formulas are covered here. Does not include with replacement/with repetition, all possible, etc. Also, this function does not account for corner cases (error checking) like if you enter a larger choose number (r) than the total number (n), etc.
math.comb and math.perm are new in version 3.8.
Combinations are the number of different ways in which objects can be arranged without regard to order.
Permutations are the number of different ways in which objects can be arranged in order.
This puzzler level is easy.
In this series we will include: data structures, algorithms, brain teasers, puzzles, and coding challenges.
The purpose of these tutorials will be to:
* Help you improve your coding skills or knock off the rust.
* Help to improve your problem solving skills.
* Help you answer questions on sites like LeetCode, Codewars, HackerRank, etc.
* Help you prepare for interviews - if needed.
* And most importantly - have fun coding by solving brain teasers and puzzles.
มุมมอง: 701
วีดีโอ
Python Puzzlers Series - Create Function to Find Factorial
มุมมอง 141ปีที่แล้ว
Welcome back everybody. Today, our python puzzler challenge is to create a function that takes in a number and returns the factorial. We will also cover using the math package factorial. A factorial is the product of an integer and all the integers below it. 5! = 5 x 4 x 3 x 2 x 1. You might be familiar with factorials as they are used in the formulas to calculate combinations and permutations....
Python Puzzlers Series - Check if String is Palindrome
มุมมอง 140ปีที่แล้ว
Today, we are going to start a new series called Python Puzzlers. In this series we will include: data structures, algorithms, brain teasers, puzzles, and coding challenges. We will start out with some easy to medium level challenges and eventually move to more difficult problems. We will consider Big O time and space later, when the puzzlers get more challenging. The purpose of these tutorials...
How to Get Microsoft Office (Excel, Word, PowerPoint) for Free
มุมมอง 867ปีที่แล้ว
Microsoft Office can be used for free at office.com if you have a Microsoft account. Get Excel for free.
Web Development Getting Started with HTML Frequently Asked Questions
มุมมอง 68ปีที่แล้ว
HTML Frequently Asked Questions Answers on GitHub: github.com/groundhogday321/HTML-Frequently-Asked-Questions In this web development tutorial we will go over the basics on how to get started with learning HTML. The following topics will be covered: What is HTML and what is it used for? What are some good resources for HTML? What browser can I use to view HTML? What are some good text editors t...
Python Plotly Dash Dashboards Introduction to Callbacks
มุมมอง 7992 ปีที่แล้ว
In this python tutorial, we will continue our plotly dash series with examples of how to use callbacks in your plotly dash dashboards. This tutorial builds on the previous dash tutorials - specifically, check out the core components tutorial and code files. Python example code on GitHub: github.com/groundhogday321/plotly-dash-examples
Python Plotly Dash Dashboards Core Components (Widgets)
มุมมอง 7792 ปีที่แล้ว
In this python tutorial, we will continue our plotly dash series with several examples of core components or widgets that you can use in your plotly dash dashboards. Python example code on GitHub: github.com/groundhogday321/plotly-dash-examples
Python Plotly Dash Dashboards Layout & Styling
มุมมอง 3.7K2 ปีที่แล้ว
In this python tutorial, we will continue our Plotly Dash series with how to layout an app or dashboard and position and style the different elements. Code on GitHub: github.com/groundhogday321/plotly-dash-examples This sample Dash dashboard shows examples of how to layout HTML, markup, a text box, a slider, a dropdown menu and some charts. For the hello world HTML we have used css to style the...
Python Plotly Dash Dashboards Hello World
มุมมอง 1.3K2 ปีที่แล้ว
In this python tutorial, we will go over how to get started with the Dash package (framework) to build interactive dashboards. Code files on GitHub: github.com/groundhogday321/plotly-dash-examples Tutorials in this Dash series will include: Hello World Layout Core Components/Widgets Basic Callbacks Interactive Dashboards More Additional Notes: To open VS code from terminal using 'code .' - Open...
Python Introduction to Plotly
มุมมอง 2K2 ปีที่แล้ว
In this python tutorial, we will go over the plotly package. Jupyter notebook on GitHub: github.com/groundhogday321/python-plotly The first part of the tutorial covers frequently asked questions (FAQs). To skip the FAQs, the tutorial starts around 7:58, however the details covered in the FAQ section can be very helpful in understanding the package. Topics covered include: plotly FAQs plotly plo...
Python openpyxl Filter, Sort, Data Validation
มุมมอง 6K2 ปีที่แล้ว
In this python tutorial, we are going to go over how to use the openpyxl package to apply data validation to spreadsheets with LibreOffice Calc. We will also briefly go over some filter and sort examples. For the examples of how to add a filter and sort condition - it is important to note that this will add the relevant instructions to the Excel file but it will not actually filter or sort the ...
Python openpyxl Conditional Formatting
มุมมอง 6K2 ปีที่แล้ว
In this python tutorial, we are going to go over how to use the openpyxl package to apply conditional formatting to spreadsheets with Excel or LibreOffice Calc. Topics covered: color scale, icon sets, data bar, highlight cells if equal to some number, highlight even numbers. openpyxl is a python library to read/write Excel files. openpyxl can help automate Excel with python (python excel tutori...
Python openpyxl Font & Cell Format Styles
มุมมอง 7K2 ปีที่แล้ว
In this python tutorial, we are going to go over how to use the openpyxl package to apply different formatting styles to spreadsheets with Excel or LibreOffice Calc. The examples we will cover include: openpyxl changing font styles, openpyxl fill cell color, openpyxl resizing cells, openpyxl changing cell border styles, and openpyxl how to change the styles of many cells all at once. openpyxl i...
Python openpyxl Charts
มุมมอง 3.9K2 ปีที่แล้ว
In this python tutorial, we are going to go over how to use the openpyxl package to create charts with Excel or LibreOffice Calc (plots with openpyxl). I made a correction to the code. It is in the Jupyter Notebook on GitHub. openpyxl is a python library to read/write Excel files. openpyxl can help automate Excel with python (python excel tutorial with openpyxl). The worksheets can be opened wi...
Python openpyxl Pandas and Numpy
มุมมอง 2.7K2 ปีที่แล้ว
In this python tutorial, we will go over the following topics: Convert a pandas dataframe to a spreadsheet with openpyxl (Pandas to Excel or LibreOffice Calc) Convert a spreadsheet to a pandas dataframe with openpyxl (Excel or LibreOffice Calc to Pandas) How to create a list or numpy array from spreadsheet column values with Excel or LibreOffice Calc. openpyxl is a python library to read/write ...
Python openpyxl Insert, Delete, Move Rows and Columns
มุมมอง 11K2 ปีที่แล้ว
Python openpyxl Insert, Delete, Move Rows and Columns
Python openpyxl Load Spreadsheet / Load Excel Workbook
มุมมอง 2.6K2 ปีที่แล้ว
Python openpyxl Load Spreadsheet / Load Excel Workbook
Python openpyxl Append, Populate, Access Data
มุมมอง 8K2 ปีที่แล้ว
Python openpyxl Append, Populate, Access Data
Python Introduction to openpyxl Using LibreOffice Calc
มุมมอง 8K2 ปีที่แล้ว
Python Introduction to openpyxl Using LibreOffice Calc
QGIS Filter Map and Export as Shapefile
มุมมอง 4.1K2 ปีที่แล้ว
QGIS Filter Map and Export as Shapefile
Python Create Word Clouds with Image Outline, Frequencies, and Interactivity
มุมมอง 1.6K2 ปีที่แล้ว
Python Create Word Clouds with Image Outline, Frequencies, and Interactivity
Python Create Package Environments with Anaconda Navigator
มุมมอง 2.7K3 ปีที่แล้ว
Python Create Package Environments with Anaconda Navigator
Python NLP Word, Sentence, Phrase Counts, Spell Check, Sentiment Analysis with TextBlob
มุมมอง 3.4K3 ปีที่แล้ว
Python NLP Word, Sentence, Phrase Counts, Spell Check, Sentiment Analysis with TextBlob
Python Sankey Diagram, Pointplot, KDE, Choropleth, Cartogram Maps with geoplot
มุมมอง 3.4K3 ปีที่แล้ว
Python Sankey Diagram, Pointplot, KDE, Choropleth, Cartogram Maps with geoplot
Swift iOS Swipe Left & Right Gestures, Swipe to New View
มุมมอง 9K3 ปีที่แล้ว
Swift iOS Swipe Left & Right Gestures, Swipe to New View
you save my day!🥰
How can you copy and paste values removing formulas ?
Thank you
Wow inner outer polygon that what I've been looking for! Thank you
Buddy, the like is very well earned (100th) because believe me the normal sort put my files like whatever XD I had to sort manually back (gladly I printed the new and old names together so I could rename them) but for future sortings it is helpful... Thanks for this tip/command! ;))
Thanks mate
Tmrw is my practicals and this video saved me! Thank you so much🥰
Fantastic video! One of the best that I have seen my life! Thank you so much!
Thank you this is like a playground for me. Plan to use this for some NOAA aircraft and mobile measurements I had done around the salt lake area.
How to find lat, long value from excel sheet of particular column containing address?
This video really helped me during my exams, so thank u bro :)
really helpful man!
OMG, you explain this better than the Develop in Swift Explorations, Fundamental books and the Gmetrix practice tests. Jeez now I can take the Swift Associate Exam with ease. Thank you.
Thank you
Welcome!
Thank you very much for the video! It's been really useful. But I have one question yet. Is it possible to integrate and show this maps on a tkinter frame, or in any other GUI library?
God bless You!
plotly dash is ridiculously powerful
Thanks allot ryan!! that's really helpful🙂
Happy to help!
Thank you so much. This is amazing
You're very welcome!
I have tried to follow along but can't figure out how to lock the reference on my Mac and don't know how you copy it down. I'll keep trying but this my brain can't get a hold of this I think.
Hi Ryan, you created an environment "python_geopandas_env". Assume in Jupyter you also need to use Numpy and Scikit-learn with geopandas to create something unique, would you add them to the "python_geopandas_env" environment as well? I have them on my PC, each in its own environment. Please advise
In theory, it should work either way - adding the other needed packages to the geopandas environment or creating a new environment with all needed packages. Usually I just try to make sure I don’t have too many packages in one environment but I would think the example you listed would work fine.
Is there a way to display dates inside the blocks in the calendar?
Thanks ❤❤
Always welcome
Thanks man
You're welcome!
thanks for this!
Welcome!
Like it.!!
Thanks!
How can I delete a formatting rule from a worksheet in a specified cell range?
Thank you!
Welcome!
how do the update functions get called?
Thank you Ryan. Very useful Video. This was exactly the things I was looking to implement. And and you figured it 2 years back 🙂
Great to hear!
This is magic to me. Thanks!
this is so cool, thank you!
You're very welcome!
thank you!
Welcome!
This is amazing, thank you so much!
Glad it was helpful!
im a beginner and im making a black jack game (so far it has everything except the ability to split). I'd like to use something like this for pretending dealer is shuffling and also to make the prompt more clear for the players (generates answers so fast people may find it confusing). Thank you so much!
Hi Ryan, it is very well explained, thank you. Can you please guide that how to save these maps as JPG or Png?
Selenium is an option but again, as EPSG of Folium is 4326 n if you want to save it along with a world file, then it is a real pain; honestly, I don’t know the solution yet but it is mandatory if you want it to be in the right projection as QGIS map layout does it automatically when you export it as a PNG.
Just a question. If i plot by Month_names and Month_num, The kde plots should be the same right?
Hello! Is it possible to create KML files in Google Colab? I've tried the same lines of code in Google Colab with the file path connecting to a gdrive folder, but the KML file is not being saved in my gdrive folder. I wonder if this is possible.
How do you do the reverse? I need cells with 0 to be blank so I can calculate an average value of few cells. If I have 0, it messes the average
I have csv file with cordinate. How can i import these cordinate in python and create polygon
is it possible to do in a regular vs code and not jupyter or does this only work with jupyter?
Thanks!
Welcome!
Thank you! This helped!! ❤❤❤
Glad it helped!
hi ryan! thank you so much for this insightful video!! very helpful
Glad it was helpful!
Thank you very much for this tutorial. It reminds me to start easier to not overwhelm oneself.
Glad it was helpful and I agree. I like to start simple and then add on little by little until I have something impressive.
impressive work, your videos are packed with useful information, like super mini courses the fact that you use notebooks helps the viewer concentrate on the subject without the lengthy typing and long pauses one thing would be also useful is timestamps in the bottom slider to quickly go through chapters, thanks a lot for your help, truly appreciated
Thanks a lot! I appreciate the feedback.
How to change back ground color while editing?
Amazing video, great value, thank you it was a lot of use to me. Finallyy someone!!!
Great to hear!
thank you!
Welcome!
thank you so much
You're welcome!