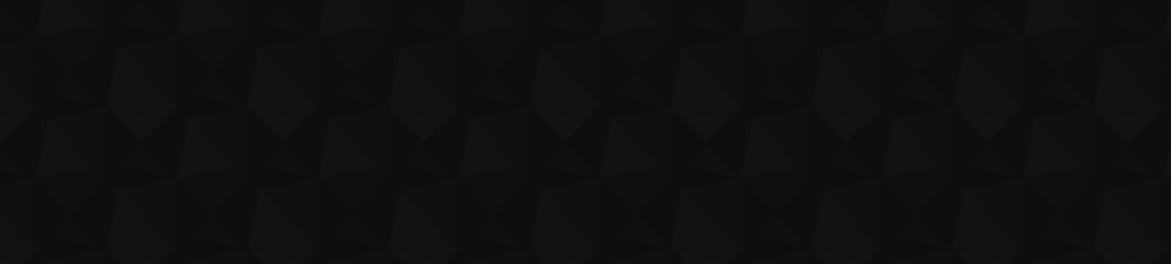
- 61
- 320 838
CodeSavant
เข้าร่วมเมื่อ 25 ธ.ค. 2014
Sort Lists Using Merge Sort
In this tutorial, we learn how to sort lists/arrays using the merge sort algorithm in Python.
มุมมอง: 741
วีดีโอ
The Multivariable Calculus Analysis of the Cobb-Douglas Production Function
มุมมอง 1.4K2 ปีที่แล้ว
The Multivariable Calculus Analysis of the Cobb-Douglas Production Function. Sources: docs.google.com/document/d/1SDXL22iqULxsJEHy0Q_K6FcqWrlHn5AJJX5H98liWRk/edit?usp=sharing Video Transcript: docs.google.com/document/d/13K4wUyKLsFiadCDz_iUcfmoGGDMlqmgoEDsUoQgYgZE/edit?usp=sharing
Create Head-Tracking Flappy Bird Game using Computer Vision in Python
มุมมอง 4.7K3 ปีที่แล้ว
This tutorial shows you how to create a Pygame-based Flappy Bird game built on OpenCV and MediaPipe that allows you to play by moving the bird with your head. Code & image files: github.com/pr28416/FlappyBirdCV
Create Rock, Paper, Scissors with Hand Gestures using OpenCV in Python
มุมมอง 5K3 ปีที่แล้ว
This tutorial shows you how to create a Rock, Paper, Scissors game where you use hand gestures to play using OpenCV in Python 3.
Ball Detection Using OpenCV in Python
มุมมอง 47K3 ปีที่แล้ว
This tutorial shows you how to detect circles, such as a ball, in a webcam capture using OpenCV in Python.
Sort Lists Using Optimized Bubble Sort in Python
มุมมอง 8483 ปีที่แล้ว
This tutorial shows you how to sort lists using an optimized variation of the bubble sort algorithm in Python 3. 0:00 Explaining the algorithm 8:00 Implementing the algorithm 9:48 Testing the algorithm
Longest Substring Without Repeating Characters in Python
มุมมอง 1.9K3 ปีที่แล้ว
This tutorial teaches you how to find and return the longest substring where every character is unique through Python 3. This can easily be modified to find the maximum length substring (rather than the substring itself). 0:00 Explaining the algorithm 10:18 Implementing the algorithm 13:02 Testing the algorithm 13:23 Variation 1: Find last longest substring without repeating characters 13:47 Va...
Split an Integer Into a List of Digits in Python
มุมมอง 13K3 ปีที่แล้ว
This tutorial shows you how to use the modulo operator and floor division to split an integer into a list of digits in Python 3. In this video, you learn to implement the algorithm to work for positive integers, and then for nonnegative integers, and finally for all integers (positive, negative, 0). This video is the new edition of an old video linked below: th-cam.com/video/c6iDh92_w9w/w-d-xo....
Balanced Brackets - Check if a string has balanced brackets in Python
มุมมอง 3.4K3 ปีที่แล้ว
This tutorial shows you how to check if a string has balanced brackets using stacks in Python 3. 0:00 Explaining the algorithm 5:21 Implementing the algorithm 8:49 Testing the algorithm
Simple Primality Test - Check if a number is prime in Python
มุมมอง 2.4K3 ปีที่แล้ว
This tutorial shows you how to determine if a number is prime in square-root time complexity using Python 3. 0:00 Implementing the algorithm 3:20 Testing the algorithm
Lower bound & upper bound binary search in Python
มุมมอง 9K3 ปีที่แล้ว
This tutorial shows you how to adapt a typical binary search algorithm so that it instead looks for the first occurrence of an element (lower bound) or index after last occurrence (upper bound). Lower and upper bound search functions are useful when trying to insert an element while maintaining a sorted order, or when searching for the number of a particular element in a sorted list. Code writt...
Prim's Algorithm - Minimum Spanning Trees in Python
มุมมอง 12K3 ปีที่แล้ว
This tutorial explains how Prim's algorithm is used to construct a minimum spanning tree of a connected undirected graph in Python 3. Be sure to like, comment, and subscribe! 0:00 Explaining the algorithm 5:00 Explaining the input 6:04 Implementing the algorithm 13:40 Testing the algorithm
Find the number of rooms in a grid using depth-first search in C++
มุมมอง 3903 ปีที่แล้ว
This tutorial shows you how to count the number of rooms (connected components) in a grid (graph) using a depth-first search in C . Be sure to like, comment, and subscribe! Adapted from CSES: cses.fi/problemset/task/1192/
Find the Number of Contiguous Subarrays With a Given Sum in Python
มุมมอง 9733 ปีที่แล้ว
This video shows you how to use prefix sums and sliding windows to count the number of subarrays with a given sum in Python 3. Be sure to like, comment, and subscribe! 0:00 Explaining the algorithm 4:52 Implementing the algorithm 7:26 Testing the algorithm Adapted from CSES: cses.fi/problemset/task/1660
Generate a Palindrome From a String in Python
มุมมอง 2.6K3 ปีที่แล้ว
This tutorial shows you how to reorder a string into a palindrome in Python 3. Be sure to like, comment, and subscribe! 0:00 Explaining the algorithm 4:17 Implementing the algorithm 8:14 Testing the algorithm Adapted from CSES: cses.fi/problemset/task/1755
Find the Maximum Subarray Sum of an Array in Python
มุมมอง 2.2K3 ปีที่แล้ว
Find the Maximum Subarray Sum of an Array in Python
Print the permutations of a list in Python
มุมมอง 7K3 ปีที่แล้ว
Print the permutations of a list in Python
Solve Sudoku using Recursive Backtracking in Python
มุมมอง 6393 ปีที่แล้ว
Solve Sudoku using Recursive Backtracking in Python
Subset Sums Variation - Number of Equal-Sum Set Bipartitions in Python
มุมมอง 1203 ปีที่แล้ว
Subset Sums Variation - Number of Equal-Sum Set Bipartitions in Python
2021 ACSL Finals Intermediate - Programming Problem 2 - King In Danger
มุมมอง 7393 ปีที่แล้ว
2021 ACSL Finals Intermediate - Programming Problem 2 - King In Danger
2021 ACSL Finals Intermediate - Programming Problem 1 - Sentences
มุมมอง 2.5K3 ปีที่แล้ว
2021 ACSL Finals Intermediate - Programming Problem 1 - Sentences
Find the maximum path sum of a triangle in Python
มุมมอง 3.3K3 ปีที่แล้ว
Find the maximum path sum of a triangle in Python
Convert prefix to infix notation in Python
มุมมอง 8593 ปีที่แล้ว
Convert prefix to infix notation in Python
Linked Lists in Python - Part 1 - Concept
มุมมอง 723 ปีที่แล้ว
Linked Lists in Python - Part 1 - Concept
Solve a maze using a breadth-first search in Python
มุมมอง 15K3 ปีที่แล้ว
Solve a maze using a breadth-first search in Python
Convert from base 10 to other bases in Python
มุมมอง 10K3 ปีที่แล้ว
Convert from base 10 to other bases in Python
Create residual plots using Google Sheets
มุมมอง 2993 ปีที่แล้ว
Create residual plots using Google Sheets
Perform linear regression on data using Google Sheets
มุมมอง 3743 ปีที่แล้ว
Perform linear regression on data using Google Sheets
Count the occurrences of characters in a string in Python
มุมมอง 10K3 ปีที่แล้ว
Count the occurrences of characters in a string in Python
thank you!
You calculate len(lst) three times -- it would be more efficient to save it in a local variable like n = len(lst) and use it instead.
This is subsequence and not subarray. Correct me if I am wrong.
pranav amesh
very hepful, I am finding a solution like this
Makes no sense - hard to understand
great video. Thanks for making this
why did you not generate sum upto the second range? I do not see the need to continue generating after our second range coz we seem not to need them
Super helpful! I'm slow, why do we minus 1? (From 14 to 13 instead?) Is it because of the 0 we added? What's so great about adding a 0 in the start? Is it customary to add it in real world code? Thanks!! :)
You are correct, it's because of the 0 we added. We added the 0 at the beginning of the sums list because the element at that 0th index in the sums list indicates the sum of elements from index 0 (inclusive) to index 0 (exclusive), but the index interval [0,0) in the original list consists of no elements. The sum of nothing is just 0, so that's what we put in that 0th index.
Thats an great way of doing it!
Thanks bro, finally understand it lol
Wow this was really useful, thanks!
In the end it's mentioned that the number of subsets are 24, that's wrong, it's 16. Since the number of subsets of a given set is 2^n, here 2^4 = 16. Other than that it's good explanation.
very good tutorial boy
Is there a resolve to do this without using even a single loop.
Came from your 2014 scratch video and your voice changed so much.
Yeah XD
My favorite part is, "Looks nice...no it doesn't actually"
Do you still make scratch tutorials? If you do can you show how to make game like flappybird or nion cat where you can move away from the area and the screen will follow you. Its hard to explain😅
Lip smacks but still thank you so much
Sorry about that, my microphone is very sensitive to the heating system's sounds (which sounds like lipsmacks). But you are welcome for the tutorial! I hoped it helped you.
STOP LIPSMACKING HDJEKDJVHEJDMCKSJDJFMEMD
Sorry about that, my microphone is very sensitive to the heating system's sounds (which sounds like lipsmacks).
@@CodeSavant lipsmack more
STOP THE LIPSMACKS I CAN HEAR THEM...
Sorry about that, my microphone is very sensitive to the heating system's sounds (which sounds like lipsmacks).
good job with this tutorial.