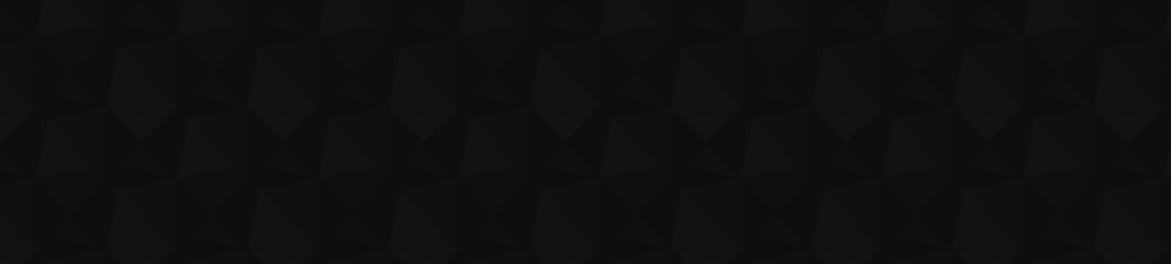
- 615
- 55 085
learningStar
Germany
เข้าร่วมเมื่อ 4 ส.ค. 2023
How to create a multiple text looking in slide using power point tutorial
Looking for a professional Looking for a professional and eye-catching PowerPoint template? Look no further! Our new template is perfect for presentations on [topic, e.g., business, education, marketing]. With its sleek design, customizable slides, and stunning visuals, you'll create presentations that stand out from the crowd. Easily replace placeholders with your own text and images to tailor the template to your specific needs. Download now and elevate your presentations to the next level!"
#PowerPointTemplate #PresentationDesign #[Topic] #Business #Education #Marketing #Designand eye-catching PowerPoint template? Look no further! Our new template is perfect for presentations on [topic, e.g., business, education, marketing]. With its sleek design, customizable slides, and stunning visuals, you'll create presentations that stand out from the crowd. Easily replace placeholders with your own text and images to tailor the template to your specific needs. Download now and elevate your presentations to the next level!"
#PowerPointTemplate #PresentationDesign #[Topic] #Business #Education #Marketing #Design
#PowerPointTemplate #PresentationDesign #[Topic] #Business #Education #Marketing #Designand eye-catching PowerPoint template? Look no further! Our new template is perfect for presentations on [topic, e.g., business, education, marketing]. With its sleek design, customizable slides, and stunning visuals, you'll create presentations that stand out from the crowd. Easily replace placeholders with your own text and images to tailor the template to your specific needs. Download now and elevate your presentations to the next level!"
#PowerPointTemplate #PresentationDesign #[Topic] #Business #Education #Marketing #Design
มุมมอง: 4
วีดีโอ
How to create a power point tutorial about WW II in power point tutorial
มุมมอง 1010 ชั่วโมงที่ผ่านมา
Looking for a professional Looking for a professional and eye-catching PowerPoint template? Look no further! Our new template is perfect for presentations on [topic, e.g., business, education, marketing]. With its sleek design, customizable slides, and stunning visuals, you'll create presentations that stand out from the crowd. Easily replace placeholders with your own text and images to tailor...
2-3 Python Functions: A def exercise part 2 ( total time until now = 29: 23)
มุมมอง 311 ชั่วโมงที่ผ่านมา
Dive into the world of Python functions with this comprehensive tutorial. Discover the power of the def keyword and learn how to create reusable blocks of code that perform specific tasks. Understand the concept of parameters, return values, and how to effectively structure your Python programs using functions. Whether you're a beginner or looking to solidify your understanding of functions, th...
How to create a level 1000 science slide in power point presentation tutorial
มุมมอง 242 ชั่วโมงที่ผ่านมา
Looking for a professional Looking for a professional and eye-catching PowerPoint template? Look no further! Our new template is perfect for presentations on [topic, e.g., business, education, marketing]. With its sleek design, customizable slides, and stunning visuals, you'll create presentations that stand out from the crowd. Easily replace placeholders with your own text and images to tailor...
How to create a great power point template about Telegram tutorial like and subscribe to support
มุมมอง 282 ชั่วโมงที่ผ่านมา
Looking for a professional Looking for a professional and eye-catching PowerPoint template? Look no further! Our new template is perfect for presentations on [topic, e.g., business, education, marketing]. With its sleek design, customizable slides, and stunning visuals, you'll create presentations that stand out from the crowd. Easily replace placeholders with your own text and images to tailor...
2-2 Python Functions: A def exercise ( total time until now = 17: 31)
มุมมอง 212 ชั่วโมงที่ผ่านมา
Dive into the world of Python functions with this comprehensive tutorial. Discover the power of the def keyword and learn how to create reusable blocks of code that perform specific tasks. Understand the concept of parameters, return values, and how to effectively structure your Python programs using functions. Whether you're a beginner or looking to solidify your understanding of functions, th...
How to create a circle transition in power point presentation tutorial like and subscribe
มุมมอง 194 ชั่วโมงที่ผ่านมา
Looking for a professional Looking for a professional and eye-catching PowerPoint template? Look no further! Our new template is perfect for presentations on [topic, e.g., business, education, marketing]. With its sleek design, customizable slides, and stunning visuals, you'll create presentations that stand out from the crowd. Easily replace placeholders with your own text and images to tailor...
How to create a triangle effect in power point presentation tutorial like and subscribe
มุมมอง 244 ชั่วโมงที่ผ่านมา
Looking for a professional Looking for a professional and eye-catching PowerPoint template? Look no further! Our new template is perfect for presentations on [topic, e.g., business, education, marketing]. With its sleek design, customizable slides, and stunning visuals, you'll create presentations that stand out from the crowd. Easily replace placeholders with your own text and images to tailor...
2-1 Python Functions: A Beginner's Guide to def ( total time until now = 9:27)
มุมมอง 144 ชั่วโมงที่ผ่านมา
Dive into the world of Python functions with this comprehensive tutorial. Discover the power of the def keyword and learn how to create reusable blocks of code that perform specific tasks. Understand the concept of parameters, return values, and how to effectively structure your Python programs using functions. Whether you're a beginner or looking to solidify your understanding of functions, th...
How to create a power point template level 100 about Berlin using crop tutorial
มุมมอง 197 ชั่วโมงที่ผ่านมา
How to create a power point template level 100 about Berlin using crop tutorial
How to create a USA power point presentation using crop and morph tutorial like and subscribe
มุมมอง 107 ชั่วโมงที่ผ่านมา
How to create a USA power point presentation using crop and morph tutorial like and subscribe
24_ Python Programming: End of the first step and what about next ( total time until now = 145:59)
มุมมอง 67 ชั่วโมงที่ผ่านมา
24_ Python Programming: End of the first step and what about next ( total time until now = 145:59)
How to make a power point presentation level 100 by using shadows tutorial
มุมมอง 189 ชั่วโมงที่ผ่านมา
How to make a power point presentation level 100 by using shadows tutorial
How to create a power point presentation trick using animation under 5 minutes tutorial
มุมมอง 259 ชั่วโมงที่ผ่านมา
How to create a power point presentation trick using animation under 5 minutes tutorial
23_ Python tutorial : first step conclusion ( total time until now = 143:06)
มุมมอง 109 ชั่วโมงที่ผ่านมา
23_ Python tutorial : first step conclusion ( total time until now = 143:06)
How to create a 4 fact power point presentation about Science for school tutorial
มุมมอง 2912 ชั่วโมงที่ผ่านมา
How to create a 4 fact power point presentation about Science for school tutorial
How to create a power point presentation about school score template tutorial
มุมมอง 1512 ชั่วโมงที่ผ่านมา
How to create a power point presentation about school score template tutorial
22_ Python Comments: A Quick Guide ( total time until now = 137:42)
มุมมอง 912 ชั่วโมงที่ผ่านมา
22_ Python Comments: A Quick Guide ( total time until now = 137:42)
How to create an amazing slide about winter in power point presentation tutorial
มุมมอง 1614 ชั่วโมงที่ผ่านมา
How to create an amazing slide about winter in power point presentation tutorial
How to create a math presentation in power point for school template tutorial
มุมมอง 1114 ชั่วโมงที่ผ่านมา
How to create a math presentation in power point for school template tutorial
21_ Python Tutorial: Create a New List of Correct Passwords ( total time until now = 132:53)
มุมมอง 814 ชั่วโมงที่ผ่านมา
21_ Python Tutorial: Create a New List of Correct Passwords ( total time until now = 132:53)
How to create an Art power point presentation template for school tutorial
มุมมอง 3416 ชั่วโมงที่ผ่านมา
How to create an Art power point presentation template for school tutorial
How to create a great power point presentation about Literature template tutorial
มุมมอง 2216 ชั่วโมงที่ผ่านมา
How to create a great power point presentation about Literature template tutorial
20_ Python Tutorial: Unlock the Power of Libraries ( total time until now = 119:09)
มุมมอง 1216 ชั่วโมงที่ผ่านมา
20_ Python Tutorial: Unlock the Power of Libraries ( total time until now = 119:09)
How to create a fire effect in power point presentation using video tutorial
มุมมอง 2519 ชั่วโมงที่ผ่านมา
How to create a fire effect in power point presentation using video tutorial
How to create a math intro power point presentation for school tutorial
มุมมอง 2019 ชั่วโมงที่ผ่านมา
How to create a math intro power point presentation for school tutorial
19_ Master List Manipulation in Python: Even and Odd Number Challenge ( total time until now=112:30)
มุมมอง 1919 ชั่วโมงที่ผ่านมา
19_ Master List Manipulation in Python: Even and Odd Number Challenge ( total time until now=112:30)
How to create a great power point presentation template under three minutes tutorial about fire
มุมมอง 4921 ชั่วโมงที่ผ่านมา
How to create a great power point presentation template under three minutes tutorial about fire
How to create a great power point presentation template under three minutes tutorial
มุมมอง 3621 ชั่วโมงที่ผ่านมา
How to create a great power point presentation template under three minutes tutorial
18_ Python List Tutorial: Master List append Divide Numbers ( total time until now =103:36)
มุมมอง 421 ชั่วโมงที่ผ่านมา
18_ Python List Tutorial: Master List append Divide Numbers ( total time until now =103:36)
Porque no me sale lo del zoom al intentar hacer eso?
i dont know but if you send your ppt file for me maybe i can fix the problem
if you dont have any way to send it too me you can upload it and put the link here for me to check and fix it
👍
beautiful
👍
Good
Excellent 👍
Good
jack = 15 james = 42 fred = 18 if jack > james : if jack > fred : print("jack is the oldest") if fred > jack : print("fred is the oldest") if james > jack : if james > fred : print("james is the oldest "
james = 42 fred = 18 jack = 15 if jack > james : if jack > fred : print("jack is the oldest") if fred > jack : print("fred is the oldest") if james > jack : if james > fred : print("james is the oldest "
james = 42 fred = 18 jack = 15 if jack > james : if jack > fred : print("jack is the oldest") if fred > jack : print("fred is the oldest") if james > jack : if james > fred : print("james is the oldest "
Good video
thanks a lot
Very good
thanks its make me happy to hear that
The answer is 220
thats right congratulations
the answer is 220
thats right congratulations
Nice I an waiting for your first session❤❤❤❤🙏🙏
Me too❤️❤️❤️
Very useful 🙏
thanks❤️❤️❤️❤️
I cant wait too❤️❤️❤️❤️
i am so happy to hear that thanks❤️❤️❤️❤️
I can't wait to see your next sessions
I am so happy to hear that thanks
Dear Sir, You have done a great job! I am very happy to see your creativity. Can you also praise my work after seeing it?🙏
جميل 🎉🎉🎉
thanks a lot
Can you provide us with a link to the template that we can use?
i will try to put the template in a telegram channel for you
@@learningStar_s i will try to put the template in a telegram channel for you
Baaad
its my pleasure to hear your opinion and i try to make my self better ❤❤❤
Amazing ❤❤ 🎉
Thanks 😄
❤️❤️❤️❤️❤️❤️
❤❤❤
Thank its help me a lot to prepare My Presentation for My class
Most welcome 😊 i am happy to help ❤❤❤
It's one of the most beautiful transitions I have ever seen ❤❤❤
thanks a lot ❤❤❤
👍 good
thanks its make me happy to hear that❤❤❤
Great
thanks its mean a lot for me
Good job
thanks its mean a lot to me
جميل ما شاء الله تبارك الله
thanks it makes me happy to hear that ❤❤❤❤
❤👏👏👏♥️♥️
thanks
Excellent creativity, stunning Presentation and Awesome Tutorial Thanks a lot.
thanks .its make me very very happy that you liked my video its my pleasure ❤❤❤❤❤❤❤
Excellent creativity, stunning Presentation and Awesome Tutorial Thanks a lot.
thanks .its make me very very happy that you liked my video its my pleasure. if you need any help i will be very happy to help you ❤❤❤
very easy but make you it leanthy tutorial. make easy
i appreciate your opinion and i will try to make them better and very happy to help
❤❤❤
thanks ❤❤❤❤
nice
I am so happy to hear that thanks❤❤❤
Why the hell do they switch sides?
its just for fun but you can change it if you want easily thanks for your comment
@@learningStar_s oh, ok then, have a nice day ❤️
@@Kompoteek thanks its make me happy that you watch my videos carefully thanks ❤❤❤
支持❤
i always appreciate your support❤❤❤
❤很精彩
i try my best to not wast your time and always thankful for your words❤❤❤
Because it's 2010 pointe and I can't find some things
i don't understand what do you want but if you want something that i can help you i will always be happy to help❤❤❤
But if you want or have to use lower version of power point i will be happy to make something that you can run it on your pc . if you want that just tell me your power point version then i try to make some template for you ppt
Any powerpoint you have
❤支持
its always make me happy to hear that that's my pleasure ❤❤❤❤
支持❤
your words make my day .❤❤❤
❤
your support mean a lot for me ❤
❤很喜欢
Your words always make me happy .
❤支持
thanks its mean a lot to me❤❤❤
No
what and why no
Great trick to use thanks
thanks its my pleasure to hear that
支持❤
thanks its always make me happy to hear that ❤❤❤❤❤
❤支持❤
thanks its means a world for me ❤❤❤❤❤💘