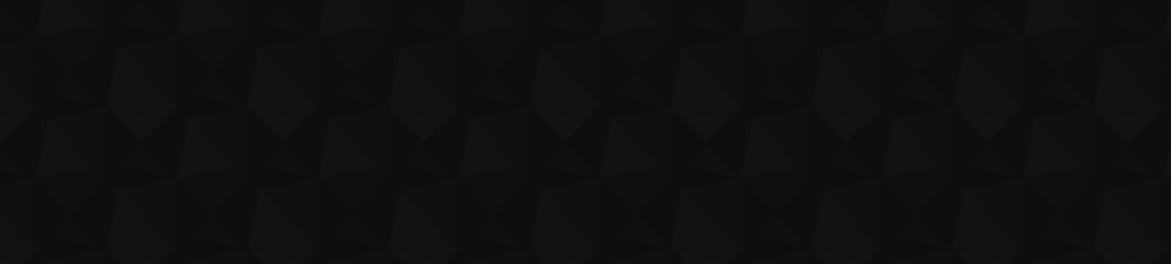
- 4
- 11 948
Sanjib Maharjan
Nepal
เข้าร่วมเมื่อ 15 พ.ค. 2022
Creating an Unbeatable TicTacToe Game Using Minimax Algorithm With Alpha-Beta Pruning in Flutter
Hello everyone, In this tutorial we're gonna build a mXn #TicTacToe game using #Flutter. You can test the final version of this game in the Play Store with this link( play.google.com/store/apps/details?id=bumble.game.tictactoe.
We'll start by developing a versus mode where 2 players can play the game alternatively. We'll then develop a versus AI mode where the players will be able to play with AI. We'll explore 3 different types of AI. The first one is a Random opponent and it is very easier to beat. As its name suggests, it selects the random move from the available one. The second one is the Thinking opponent which is a bit trickier than the first opponent. The last one is the Smart Opponent, which uses a #minimax algorithm to find its next move. This opponent is impossible to beat(at least the 3X3 variant), we can either lose or in the best case draw the game. We'll also use #AlphaBetaPruning to optimize the minimax algorithm. Finally, we'll develop an AI vs AI simulation where 2 AI from the 3 opponents(inclusive) are chosen randomly and a match is initiated between them.
Tic Tac Toe is one of the old-school pen-and-paper games that most of us have played as a child. I remember playing with friends by drawing all over our benches and textbooks while our teacher was still teaching. The benches used to be full of tictactoe boxes and all of us used to have tricks of our own to beat one another. In this tutorial, we'll have a closer look at that game and build it using Flutter Casual Games Toolkit.
00:00 Intro
02:20 Coding Start
04:24 Designing TicTacToe Board
06:42 Custom Painter
09:01 Winning Lines
11:16 Board State(Main logic)
14:08 Change Notifier
17:01 Use Board State in Game
18:25 TicTacToe Winning Logic
25:43 Versus Mode
27:03 AI Opponent Class
27:15 Random Opponent
30:24 Thinking Opponent(Logical Opponent)
32:23 3X3 TicTacToe Breakdown
33:43 Minimax Algorithm
35:24 Minimax Tree
36:12 TicTacToe Game in Minimax Tree
37:08 Smart Opponent(Minimax AI Opponent)
41:46 Optimization Check for Smart Opponent
42:03 Alpha Beta Pruning(Smart AI Optimization)
42:45 Alpha Beta Comparision
43:15 Winning Logic Bug and Fix
45:02 Modified Game(New UI and some changes)
45:11 AI VS AI Simulation
► Test the Game in Playstore:
play.google.com/store/apps/details?id=bumble.game.tictactoe
► Written Tutorial:
cshanjib.medium.com/creating-an-unbeatable-tic-tac-toe-game-using-minimax-algorithm-with-alpha-beta-pruning-in-flutter-f666594be0b4
► Starter Repo:
github.com/cshanjib/tictactoe_flutter/tree/starter
► Completed Repo:
github.com/cshanjib/tictactoe_flutter/tree/main
► Updated Repo:
github.com/cshanjib/tictactoe_flutter/tree/game
►Flutter Game: flutter.dev/games
►Flutter Game Template: github.com/flutter/samples/tree/master/game_template
►Game Reference:
github.com/filiph/tictactoe
My other Tutorials:
► Flutter Version Management(fvm) Tutorial
th-cam.com/video/4Ph7nK7rG_0/w-d-xo.html
cshanjib.medium.com/setting-up-fvm-flutter-version-management-properly-ab45ade0dd55
► Login and Register Design Tutorial With Form Validation
th-cam.com/video/2QK79dM3Gdg/w-d-xo.html
cshanjib.medium.com/flutter-responsive-design-tutorial-login-and-registration-in-a-dialog-with-form-validation-4a4c65786f77
► Dependency Injection Tutorial
cshanjib.medium.com/dependency-injection-using-injector-and-getit-packages-in-flutter-c3739c2ad5dd
► Responsive Design Tutorial
cshanjib.medium.com/responsive-ui-in-a-flutter-application-193c126306f6
► Music: Bensound.com
► Let's Connect
Medium: medium.com/@cshanjib
GitHub: github.com/cshanjib
LinkedIn: np.linkedin.com/in/sanjib-maharjan-1108a8a6
Instagram: cshanjib.me
#tictactoe #minimax #alpha_beta_pruning #flutter #mxn_tictactoe
We'll start by developing a versus mode where 2 players can play the game alternatively. We'll then develop a versus AI mode where the players will be able to play with AI. We'll explore 3 different types of AI. The first one is a Random opponent and it is very easier to beat. As its name suggests, it selects the random move from the available one. The second one is the Thinking opponent which is a bit trickier than the first opponent. The last one is the Smart Opponent, which uses a #minimax algorithm to find its next move. This opponent is impossible to beat(at least the 3X3 variant), we can either lose or in the best case draw the game. We'll also use #AlphaBetaPruning to optimize the minimax algorithm. Finally, we'll develop an AI vs AI simulation where 2 AI from the 3 opponents(inclusive) are chosen randomly and a match is initiated between them.
Tic Tac Toe is one of the old-school pen-and-paper games that most of us have played as a child. I remember playing with friends by drawing all over our benches and textbooks while our teacher was still teaching. The benches used to be full of tictactoe boxes and all of us used to have tricks of our own to beat one another. In this tutorial, we'll have a closer look at that game and build it using Flutter Casual Games Toolkit.
00:00 Intro
02:20 Coding Start
04:24 Designing TicTacToe Board
06:42 Custom Painter
09:01 Winning Lines
11:16 Board State(Main logic)
14:08 Change Notifier
17:01 Use Board State in Game
18:25 TicTacToe Winning Logic
25:43 Versus Mode
27:03 AI Opponent Class
27:15 Random Opponent
30:24 Thinking Opponent(Logical Opponent)
32:23 3X3 TicTacToe Breakdown
33:43 Minimax Algorithm
35:24 Minimax Tree
36:12 TicTacToe Game in Minimax Tree
37:08 Smart Opponent(Minimax AI Opponent)
41:46 Optimization Check for Smart Opponent
42:03 Alpha Beta Pruning(Smart AI Optimization)
42:45 Alpha Beta Comparision
43:15 Winning Logic Bug and Fix
45:02 Modified Game(New UI and some changes)
45:11 AI VS AI Simulation
► Test the Game in Playstore:
play.google.com/store/apps/details?id=bumble.game.tictactoe
► Written Tutorial:
cshanjib.medium.com/creating-an-unbeatable-tic-tac-toe-game-using-minimax-algorithm-with-alpha-beta-pruning-in-flutter-f666594be0b4
► Starter Repo:
github.com/cshanjib/tictactoe_flutter/tree/starter
► Completed Repo:
github.com/cshanjib/tictactoe_flutter/tree/main
► Updated Repo:
github.com/cshanjib/tictactoe_flutter/tree/game
►Flutter Game: flutter.dev/games
►Flutter Game Template: github.com/flutter/samples/tree/master/game_template
►Game Reference:
github.com/filiph/tictactoe
My other Tutorials:
► Flutter Version Management(fvm) Tutorial
th-cam.com/video/4Ph7nK7rG_0/w-d-xo.html
cshanjib.medium.com/setting-up-fvm-flutter-version-management-properly-ab45ade0dd55
► Login and Register Design Tutorial With Form Validation
th-cam.com/video/2QK79dM3Gdg/w-d-xo.html
cshanjib.medium.com/flutter-responsive-design-tutorial-login-and-registration-in-a-dialog-with-form-validation-4a4c65786f77
► Dependency Injection Tutorial
cshanjib.medium.com/dependency-injection-using-injector-and-getit-packages-in-flutter-c3739c2ad5dd
► Responsive Design Tutorial
cshanjib.medium.com/responsive-ui-in-a-flutter-application-193c126306f6
► Music: Bensound.com
► Let's Connect
Medium: medium.com/@cshanjib
GitHub: github.com/cshanjib
LinkedIn: np.linkedin.com/in/sanjib-maharjan-1108a8a6
Instagram: cshanjib.me
#tictactoe #minimax #alpha_beta_pruning #flutter #mxn_tictactoe
มุมมอง: 610
วีดีโอ
Pull to Refresh in Flutter | RefreshIndicator | CupertinoSliverRefreshControl
มุมมอง 3.7Kปีที่แล้ว
#PullToRefresh is a touchscreen gesture that involves the user pulling down a list to trigger an event to fetch the most recent data. This feature can enhance the mobile app by allowing the user to load the most recent data without needing to restart the application. Popular apps like Facebook, Instagram, etc. also have them to load the most recent post. In Flutter we can implement the pull-to-...
Responsive Login & Registration Form In Flutter with Form Validation
มุมมอง 2.3K2 ปีที่แล้ว
Responsive Login & Registration Form In Flutter with Form Validation In this #flutter tutorial, we'll design the #responsive #login and #register form in a popup with form validation. In this tutorial, we're also gonna cover topics like custom painter and clipper, MediaQuery, etc. 00:00 Intro 01:01 Project Setup 04:31 Creating Dialogs in flutter 05:57 "No MaterialLocalizations found" issue, exp...
FVM - Flutter Version Management: Managing Multiple Versions of Flutter
มุมมอง 5K2 ปีที่แล้ว
Managing different versions is one of the important topics in software development. Normally there can only be one version of Flutter at a time and switching the versions is quite tricky and also time-consuming since it downloads the SDK and libraries from the beginning even though we had used that version earlier before using the current version. Hence If we are working on more than one projec...
I just wanted to take a moment to appreciate how fantastic this video is. It's well-edited and presented in a way that makes it easy for learners to grasp many concepts. Thanks a lot! Keep them coming.
Very Helpful and Great Content.
15:16 - what if you run flutter --version in the terminal ? It will show your global version there I think not the one you used with fvm use
Well, it all depends on the global path that you have set in your system. This is explained here at 13:08. If you have installed flutter in 2 different ways(ie normal way and then also fvm way), then you probably have the flutter path set to the normal flutter path. If you want to use the global version from fvm correctly, then you must update the environment variable path to “/User/<username>/fvm/default/bin”(this path is for Macos, update the path according to your platform). If you do this, then your system will take the global version set in fvm from anywhere in the system. ie(flutter -version will give the global version set in fvm and fvm flutter -version will give the projects set version if any)
@@cshanjib I understand thanks for confirming. Thought the same, so whatever we have in fvm is only for android studio/vs code but not for the terminal. If you use Android-Studio terminal flutter command it will always use whatever version is exported in the path but during builds and commands run through studio will use the linked .fvm/flutter_bin
I love this, thank you so much
good bro
really a great tutorial, It was vert helpful.
This guy is genius
Nice video. Thank you.
great!
can you make videos explaining these different notifiers and other packages related, your method of teaching is really nice with the animations great videoa!
Sure. Will try to upload those videos.
nice very informative
Great !!! Keep it up Sanjiv
Nice Bro!
P r o m o s m 👊
Great tutorial 👏👏
Very nice and clear explanation. Thanks Sir. Keep share
Really nice video, thank you.
What if i want to run the project using F5 in vscode. does it run the command with fvm? if not, how can i add fvm prefix to the F5 action ?
Assuming that you had set the flutter version correctly using the steps mentioned in the video, VSCode (and also Android studio) will use that set version while running the project either we use F5 or click the play button (in the IDE). No extra configuration is needed.
very helpful keep going